Recursion in C Language
0 297
Functions may be used recursively that is, a function may call itself either directly or indirectly.
Recursive code is more compact and often much easier to write and understand.
Recursion involves a calling function within itself which leads to a call stack.
Types of recursion
1Direct recursion
2Indirect recursion
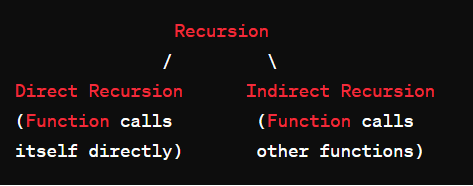
Direct recursion
The function itself calls directly is called direct recursion.
Only one function is called by itself. Direct recursion makes overhead.
This function is called by the same function. In direct function when the value is called next time, the value of the local variable is stored.
Direct function engaged memory location.
Indirect recursion
The function itself calls indirectly is called Indirect recursion.
More than one function is called by the other function many times.
Indirect recursion does not make any overhead as direct recursion.
While the indirect function is called by another function Value will automatically lost when any other function is called.
A local variable of indirect function is not engaged.
Example:
// Program for recursion in C #include<stdio.h>// Function prototype for total function int total(int i); int main() { int result = total(10); // Call total function with argument 10 printf("%d", result); // Print the result return 0; // Return 0 to indicate successful execution } // Recursive function to calculate the sum of numbers from 1 to i int total(int i) { if (i > 0) // Base case: if i is greater than 0 { return i + total(i - 1); // Return i + sum of numbers from 1 to i-1 } else { return 0; // Return 0 when i becomes 0 } }
Output:
55

Share:
Comments
Waiting for your comments