Size of operator in C
0 140
The sizeof() operator in C identifies the size in bytes of the data type or variable.
It allocates memory dynamically, works with arrays, and understands the storage requirements of different types.
sizeof() operator in C operates on a single operand so it is known as the Unary operator.
sizeof() in C acts like a byte ruler, swiftly measuring the memory space a variable or data type occupies.
The output of sizeof() operator is an unsigned integer of type size_t.
This represents the size of the operand in bytes.
Sizeof() operator Syntax
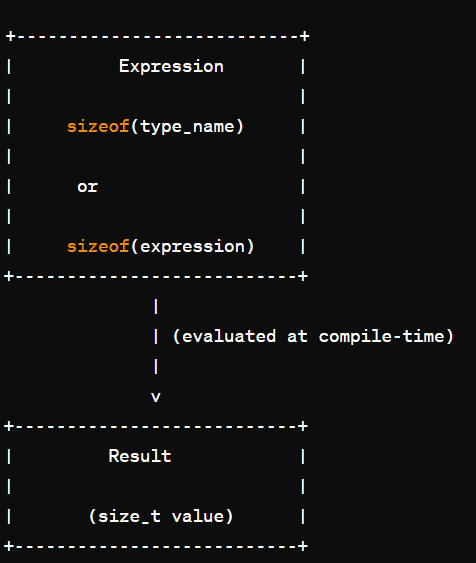
Types and Uses of sizeof() operator in C
1Identifying the size of Data Types
In C, sizeof() acts as a handy tool to figure out the memory size of any data type, like a quick peek at the space it takes up in memory.
For example:
sizeof() int
this will give you the size of the integer.
sizeof()char
this will give you the size of the character.
2 Identifying the size of the Variable
We can use sizeof to identify how much memory-specific variable occupies.
For example:
sizeof() variableThis function helps you find out how many elements exist in an array.
3Dynamic memory allocation
It helps in dynamically assigning memory using functions like malloc and calloc, giving us the flexibility to allocate memory as needed.
4Portability
The size of a data type can change depending on the platform and compiler you're using.
5Array sizes
When working with arrays, sizeof() can be used to determine the size of the entire array in bytes.
For example:
sizeof(array) / sizeof(array[0])
This function helps you find out how many elements exist in an array.
6Structure and unions
When you're working with structures and unions, the operator tells you how much memory the entire structure or union occupies, considering any extra space (padding) it might need.
The sizeof() operator in C allows us to find out how much space different things like data types, variables, arrays, and structures take up in memory.
Program for sizeof() operator in C
#include<stdio.h>
int main()
{
int A;
printf("%d\n", sizeof(A));
printf("%d\n", sizeof(int));
printf("%d\n", sizeof(4));
printf("%d\n", sizeof(char));
printf("%d\n", sizeof(float));
printf("%d\n", sizeof(double));
return 0;
}
Output:
4
4
4
1
4
8

Share:
Comments
Waiting for your comments