Types of Errors in C Programming
0 389
Programming errors in C can be tricky, but understanding them is key to becoming a better programmer.
C programming errors are sorted into various types under different labels.
1 Syntax errors
2 Semantic errors
3 Logical errors
4 Runtime errors
5 Memory errors
Types of Errors in C Programming
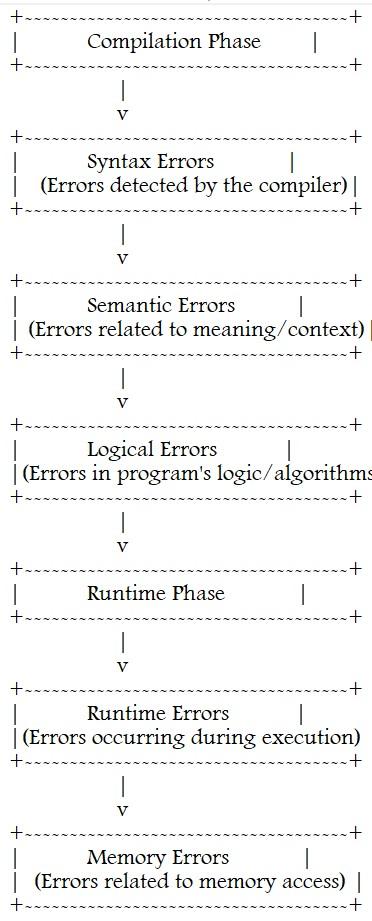
Syntax Errors
Syntax errors are the most common errors and occur when the rules of the programming language are broken.
It means you've written something that doesn't make sense to the compiler.
Syntax errors are usually easy to spot because the compiler will typically point them out with an error message.
Example:
int x=80; printf("hello c programming") // missing semicolon at the end of line.
Semantic Errors
Semantic errors occur when the syntax of the program is correct, but the statements are not logically sound.
The code will compile without errors, but it may not produce the desired output or may produce unexpected results.
Example:
int A = 20; float B = 5; int C=A/B;If you're trying to divide a number that's supposed to be whole (like an integer) by a number that can have decimal parts (like a float), it might not give you the result you expect.
that's because the computer might not handle the numbers exactly as you think it would.
Logical errors
Logical errors are the trickiest errors to find because the code will compile without any errors, and it will run without crashing.
However, the output will not be what was expected because there's a mistake in the algorithm or the logic of the program.
Example:
#include<stdio.h>int main() { int A= 30; if (A> 50) { printf("A is greater than 50\n"); } else { printf(" A is not greater than 10\n"); // Logical error: A is not greater than 50, but the message says. }
Runtime errors
A Runtime errors happens when you run your program and something goes wrong while it's running.
It's like when you're playing a game and it suddenly crashes, but if the user enters '0' as the second number, you can't divide by zero.
This would cause a runtime error because the program encounters a problem while it's running.
Example:
#include<stdio.h> int main() { int A, B; float result; printf("Enter two numbers: "); scanf("%d %d", &A, &B);//Check if B is zero before dividing if (B != 0) { result = A / B; printf("Result of division: %.2f\n", result); } else { printf("Error: Cannot divide by zero!\n"); } return 0; }Output:
Enter two numbers: 4 2 Result of division: 2.00If the user enters 0 as the second number, the program will crash with a runtime error because you can't divide by zero.
Memory errors
Memory errors occur when there are issues related to Memory Allocation and Deallocation.
If you try to access memory that your program hasn't explicitly reserved, it can lead to unpredictable behavior or crashes.
Example:
#include <stdio.h> int main() { int A; printf("Value of A: %d\n", A); // Accessing uninitialized variable return 0; }
Output:
Value of A: 0

Share:
Comments
Waiting for your comments