C Tokens in C Language
0 696
In C language C tokens are the Smallest Units of a Program.
They are categorized into different types.
1 Keywords in C
2 Identifiers in C
3 Constants in C
4 String Literals in C
5 Operators in C
6 Punctuation Symbols in C
Different types of Tokens in C
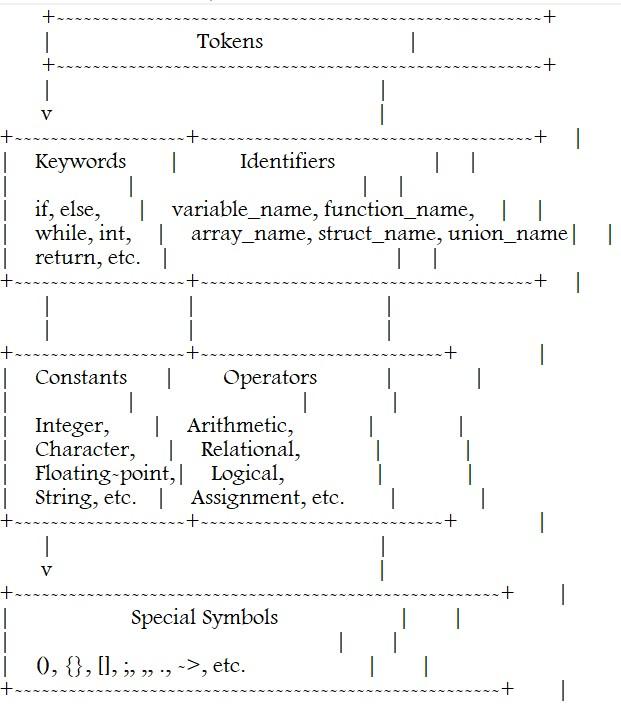
Keywords in C
keywords like main, return are reserved words with predefined meanings.
For example:
int main(){ return 0; }
Identifiers in C
Identifiers such as int, char, float are names given to various program elements.
For example:
int age=45;
Constants in C
Constants are Fixed values used in the program.
For example:
const float pi=3.14;
String Literals in C
String Literals are Sequence of characters closed in double-quotes.
For example:
char *greeting = "good morning world";
*greeting points to the location where the string 'good morning world' is stored in memory.
Operators in C
Operators are Symbols used to perform different operations.
For example:
int total=12+3;
Punctuation Symbols in C
Punctuation Symbols are Characters used for Syntax and Structure.
operators like +, -, *, /, =, ==, !=, <, >, <=, >=, &&, ||, etc., are essential punctuation symbols in C.
For example:
if(condition){ printf("True") }Each tokens have a specific purpose and they contribute to the structure and functionality of the C program.
C Program for C tokens
#include<stdio.h>Output:int main() { int n = 23; int m = 67; int sum = n + m; if(sum > 10) { printf("sum is greater than 10\n"); } else { printf("sum is not greater than 10\n"); } for(int i = 0; i < 5; i++) { printf("Iteration %d\n", i); } printf("The sum is : %d", sum); return 0; }
sum is greater than 10 Iteration 0 Iteration 1 Iteration 2 Iteration 3 Iteration 4 The sum is: 90

Share:
Comments
Waiting for your comments